제가 작성할 로그인 구현 설명은
백엔드로 Spring Boot, Spring Data JPA 를,
프론트엔드로 React.js를 사용합니다.
1. 백엔드
application.yml
DB와 JPA 사용을 위한 yml 설정을 해주었습니다.
spring:
profiles:
include: oauth
datasource:
driverClassName: org.h2.Driver
password: password
url: jdbc:h2:mem:testdb;MODE=Mysql;
username: sa
h2:
console:
enabled: true
path: /h2-console
jpa:
database-platform: org.hibernate.dialect.MariaDB103Dialect
hibernate:
ddl-auto: create
properties:
hibernate:
format_sql: true
show-sql: true
CORS 설정
프론트엔드는 http://localhost:3000, 백엔드는 http://localhost:8080 을 사용할것이기 때문에
CORS 설정을 해주었습니다.
package com.yelim.security.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:3000")
.allowedMethods("GET", "POST", "PUT", "DELETE")
.exposedHeaders("location")
.allowedHeaders("*")
.allowCredentials(true);
}
}
User
package com.yelim.security.domain;
import com.sun.istack.NotNull;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import lombok.Getter;
@Entity
@Getter
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String email;
@NotNull
private String password;
public User() {}
public User(Long id, String email, String password) {
this.id = id;
this.email = email;
this.password = password;
}
}
AuthController
로그인과 회원가입 api 를 껍데기만 마련해뒀습니다.
@RestController
public class AuthController {
@PostMapping("/sign-up")
public ResponseEntity<String> signUp() {
return ResponseEntity.ok("회원가입 완료");
}
@GetMapping("/sign-in")
public ResponseEntity<String> signIn() {
return ResponseEntity.ok("로그인 완료");
}
}
UserController
내 정보 조회 기능을 껍데기만 마련해두었습니다.
package com.yelim.security.controller;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@GetMapping("/users/me")
public ResponseEntity<String> findMe() {
return ResponseEntity.ok("me!");
}
}
2. 프론트엔드
MyPage.js
내 정보 조회 결과를 보여줄 페이지입니다.
import React, {useEffect, useState} from 'react';
import findMe from "../api/FindMe";
const MyPage = () => {
const [result, setResult] = useState("");
useEffect(() => {
findMe().then(resultPromise => setResult(resultPromise));
}, []);
return (
<>
{result}
</>
);
};
export default MyPage;
SignIn.js
로그인 페이지입니다.
import React from 'react';
const SignIn = () => {
return (
<div>
<input type="text" placeholder="사용자이름"/>
<input type="password" placeholder="비밀번호"/>
<button>sign in</button>
</div>
);
};
export default SignIn;
App.js
import React from "react";
import {BrowserRouter as Router, Route, Switch} from "react-router-dom";
import SignIn from "./sign-in/SignIn";
import MyPage from "./my-page/MyPage";
const App = () => {
return (
<Router>
<Switch>
<Route path="/sign-in" exact component={SignIn}/>
<Route path="/" exact component={MyPage}/>
</Switch>
</Router>
);
}
export default App;
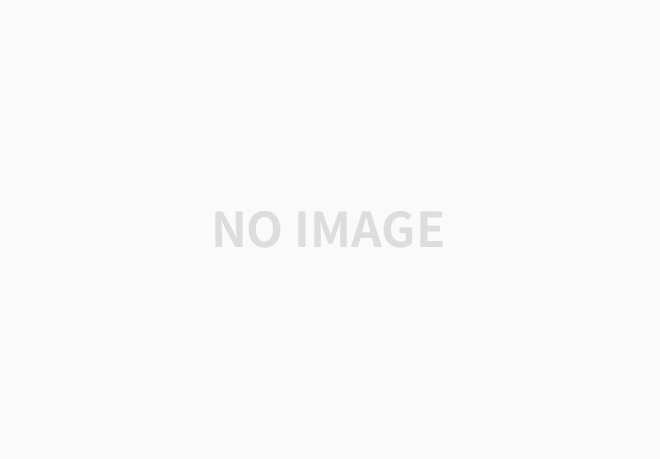
'Spring Security' 카테고리의 다른 글
소셜로그인 6. 커스텀 OAuth2UserService 만들기 (1) | 2021.09.06 |
---|---|
소셜로그인 5. UserDetails, OAuth2User 타입 객체 만들기 (0) | 2021.09.05 |
소셜로그인 4. 토큰 정보 정의 (0) | 2021.09.05 |
소셜로그인 3. Spring Security 의존성 및 yml (0) | 2021.09.05 |
소셜로그인 2. 구글 콘솔에서 Oauth 앱 생성하기 - 승인된 리다이렉션 URI란 (1) | 2021.09.05 |